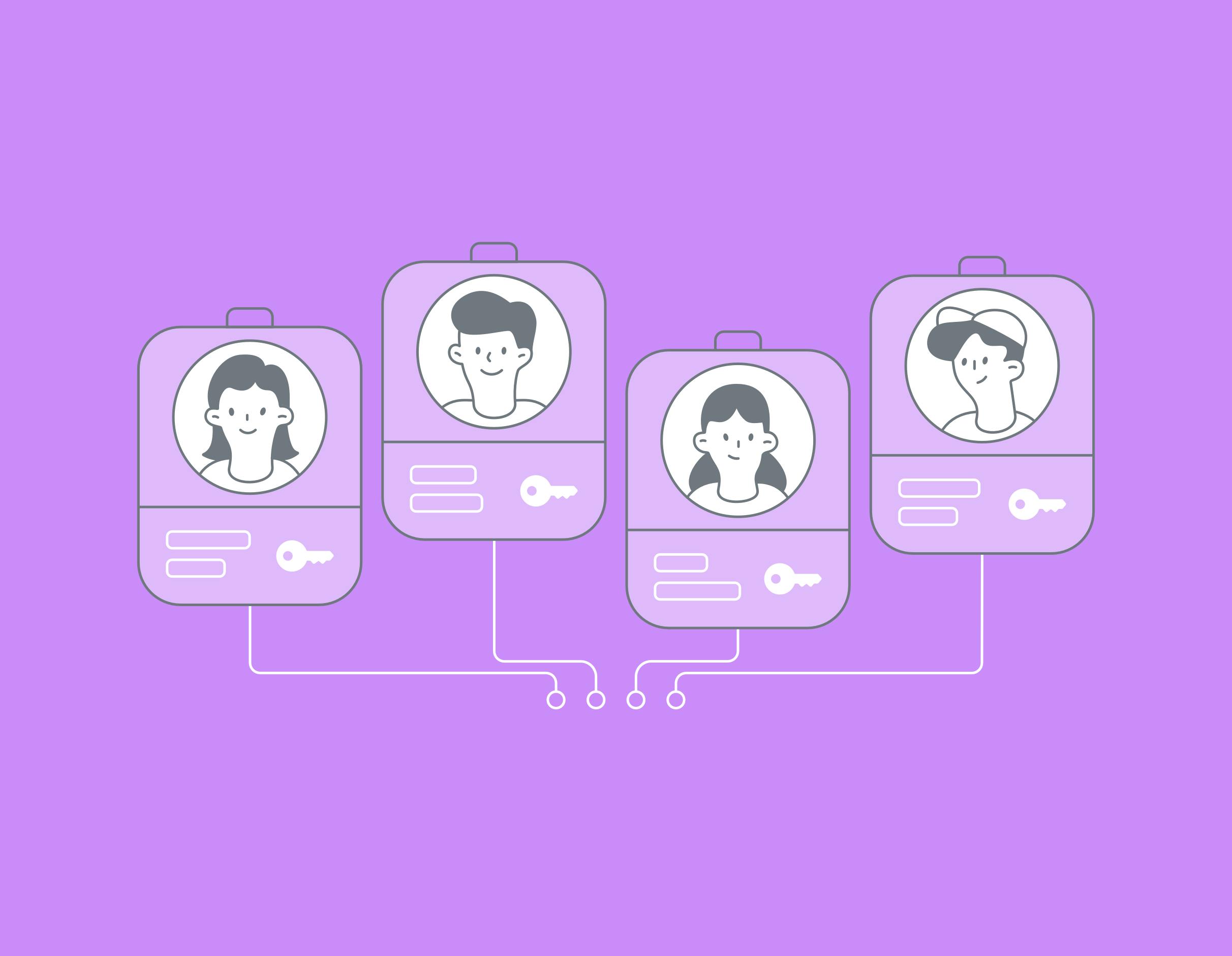
Explore software distribution: parties involved, optimizing delivery, copy protection, and security. Learn how to benefit developers and end-users.
Java compiles code into byte code, which can then be read back using a full Java bytecode. Although Java decompilers are often not 100% accurate, they're accurate enough to make it a security concern for many.
If you want to be protected from having your program's code being stolen, you can obfuscate your code.
This is a process done during compilation by creating a separate program that makes your compiled code not humanly readable while having identical functionality.
This way, if someone decompiles one of your Java programs, they won't have the source code.
Developers can protect Java source code through various techniques, including obfuscation, encryption, secure socket layer connections and certificate verifications, and other security measures.
Here are the general pros and cons of these approaches:
It's important to note that while these measures can provide additional layers and methods of protection, they should not be considered as standalone protection solutions.
A holistic approach to application protection, including the usage of secure coding practices, proper access controls, input validation methods, and regular security updates, should be implemented to ensure comprehensive protection against various threats.
Obfuscation refers to the practice of intentionally making code more difficult to understand or reverse-engineer.
The primary goal of obfuscation is to obscure the code's logic and structure while preserving its functionality. This is done to be protected from unauthorized access, theft, or tampering.
Obfuscation techniques involve transforming the code in various ways to make it less readable to humans while keeping it executable by computers.
Here are a few common methods used in code obfuscation:
We wrote a sample project demonstrating the usage of LicenseSpring in creating a Java application.
The project utilizes the LicenseSpring License Client library to manage user license activation, checking, and deactivation.
To build the project, run the following command in the project's root directory:
"mvn clean package"
This will generate a JAR file with dependencies and obfuscate the code using ProGuard. The resulting JAR file will be located in the target directory.
Run the application using the command line, providing the required arguments:
"java -jar target/licensespring-obfuscation-sample-1.0-SNAPSHOT-proguarded.jar <apiKey> <sharedKey> <licenceKey> <productName> <isStaging>"
Replace <apiKey>, <sharedKey>, <licenceKey>, <productName>, and <isStaging> with the appropriate values for your LicenseSpring account and product. Use "yes" for <isStaging> if you want to use the staging API, otherwise use "no".
In our code sample, we use ProGuard to obfuscate the code.
ProGuard is an open-source command-line tool that shrinks, optimizes, and obfuscates Java code. It can optimize bytecode as well as detect and remove unused instructions.
ProGuard is free software and is distributed under the GNU General Public License, version 2.
More information about ProGuard can be found on Guardsquare's ProGuard Page.
AppShield is a security solution created by Promon that protects mobile and desktop software against various types and methods of attacks.
It offers features like obfuscation, shielding, and runtime protection.
It aims to prevent reverse engineering, tampering, and other malicious activities.
Protector4J is a bytecode Java obfuscator and encryptor.
It provides obfuscation and encryption features for Java code to be protected from reverse engineering, decompilation, and tampering.
Its primary goal is to make it more difficult for attackers to understand, modify, or reuse the source code.
Wibu-Systems is a company that offers software protection system solutions, and one such example of their products is CodeMeter, which protects various Java software applications.
CodeMeter offers security features like encryption, various licensing mechanisms, and secure execution methods to provide protection for Java software from common attacks.
In this scenario, the applications are hosted on the server side and shared among multiple users or tenants. The server-side application is responsible for interacting with the LicenseSpring licensing API.
When it comes to API key management, it's crucial to prioritize security.
One approach is to securely store the API key on the server.
This can be achieved by utilizing environment variables, which are not exposed in the source java code and can be changed without modifying the application.
Another option is to store the API key in a secure configuration file that the server application reads from.
To ensure the secure storage and protection of sensitive information, such as API keys, encryption should be employed for both storage and transmission.
Server-side applications can leverage solutions like AWS Secrets Manager or HashiCorp's Vault to effectively manage and retrieve API keys securely.
These services offer robust security tools and measures to provide protection of sensitive data from unauthorized access.
In this scenario, the applications are installed and executed directly on the client's devices. These applications require interaction with the LicenseSpring licensing API to verify license validity, which may occur on each startup or at regular intervals.
The API key bundled with the client Java class and application files is protected by employing obfuscation techniques is recommended. Obfuscation helps deter casual attempts to retrieve the key.
Secure storage of the API key depends on the platform being used. For example, on Windows, the Data Protection API (DPAPI) can be utilized for secure storage. Other platforms, like Linux, may offer their secure storage APIs or services, which can be employed to safeguard the API key.
It is important to note that in client-installed applications, the threat model differs from server-side scenarios.
Anything stored on the client's device or in the client's memory may potentially be accessed by the client.
Therefore, it is crucial to understand that no method can completely prevent a determined attacker from such attacks, such as retrieving the API key.
The strategies mentioned here primarily aim to make it more difficult for unauthorized individuals to access the key, rather than attempting to restrict it entirely.
The LicenseSpring Java SDK includes a pre-installed public key certificate that corresponds to the private key held on the LicenseSpring APIs' servers.
This certificate plays a crucial role in establishing a trusted communication channel between your application and LicenseSpring's server.
Whenever the LicenseSpring Java SDK interacts with the LicenseSpring APIs, the communication process incorporates a mechanism to ensure the authenticity and integrity of the received response.
The servers utilize their private key to sign each response before sending it back to the client.
These digital signatures act as a seal, providing a high level of confidence that the response data has not been tampered with during transmission and that it originated from the LicenseSpring servers.
Upon receiving a response from the LicenseSpring APIs, the Java SDK performs a vital verification step.
It utilizes the pre-installed public key certificate to validate the digital signature attached to the response.
This verification process confirms that the response does indeed originate from the LicenseSpring APIs and that it has remained unaltered since it was signed.
By employing this process of signing and verifying responses, a secure communication channel is established between your Java application and LicenseSpring.
Even in the presence of potential attackers, the integrity and authenticity of the server responses can be reliably confirmed, thereby ensuring the security and trustworthiness of your application's licensing operations.
Protecting a Java application in a virtual machine involves the usage of various additional protection measures to safeguard the application code and sensitive data from unauthorized access or tampering.
Here are some key virtual machine-specific strategies to enhance the protection of Java applications: